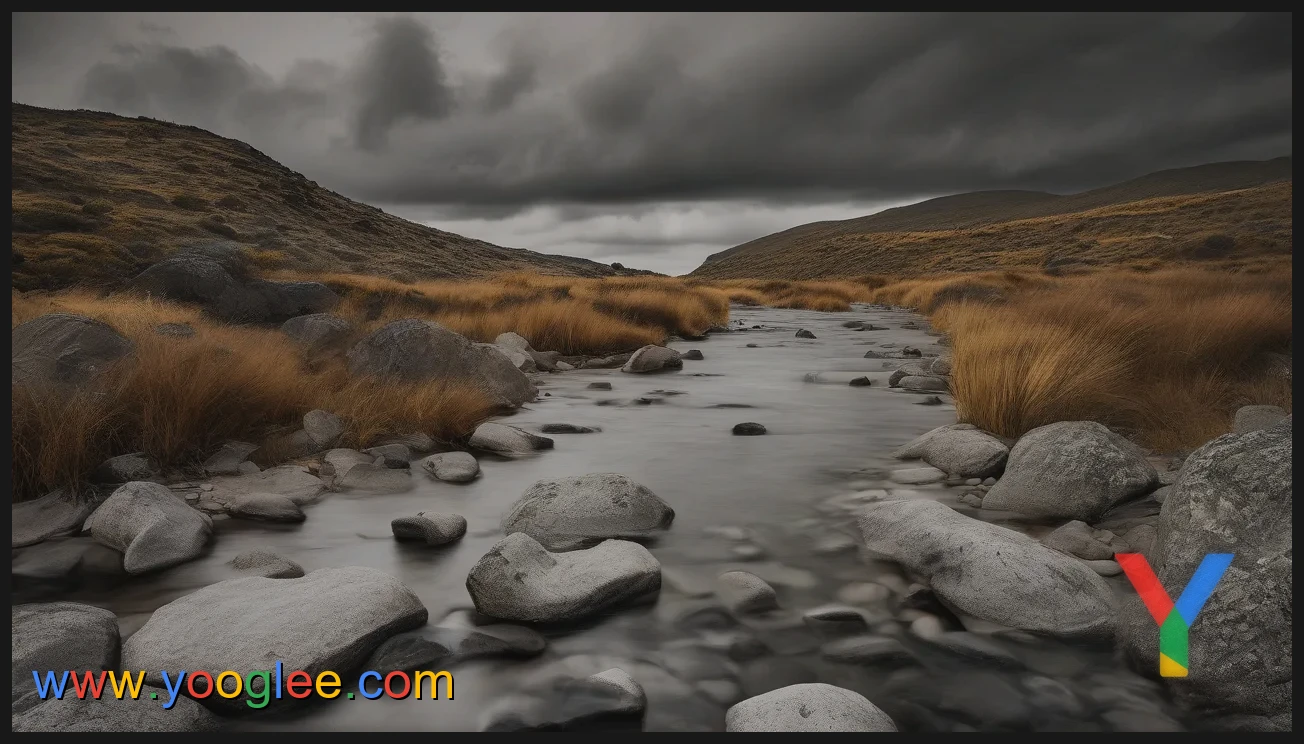
Naive Bayes with Scikit-Learn
Published on 4/19/2025 • 5 min read
Implementation of Naive Bayes in Scikit-learn
Naive Bayes is a popular machine learning algorithm known for its simplicity and effectiveness in classification tasks. In particular, the implementation of Naive Bayes in the Scikit-learn library provides a powerful tool for building and training classification models. By leveraging the probabilistic principles of Bayes' theorem and assuming independence among features, Naive Bayes in Scikit-learn offers a straightforward yet highly accurate approach to classifying data. In this introductory guide, we will explore the fundamentals of Naive Bayes in Scikit-learn, its advantages, and how to implement it in various classification tasks.
Naive Bayes is a popular machine learning algorithm that is commonly used for classification tasks. It is based on Bayes' theorem, which calculates the probability of a hypothesis given the evidence. In the context of machine learning, Naive Bayes assumes that the features are independent of each other, which is why it is called naive. Scikit-learn is a powerful machine learning library in Python that provides implementations of various machine learning algorithms, including Naive Bayes. The Naive Bayes implementation in scikit-learn is easy to use and efficient, making it a popular choice for many data scientists and machine learning practitioners. To use Naive Bayes in scikit-learn, you first need to import the necessary modules: \`\`\`python from sklearn.naive_bayes import GaussianNB from sklearn.model_selection import train_test_split from sklearn.metrics import accuracy_score \`\`\` Next, you need to load your dataset and split it into training and testing sets: \`\`\`python X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42) \`\`\` Then, you can create an instance of the Naive Bayes classifier and train it on the training data: \`\`\`python nb = GaussianNB() nb.fit(X_train, y_train) \`\`\` Finally, you can make predictions on the test data and evaluate the performance of the model: \`\`\`python y_pred = nb
Benefits of Naive Bayes with Scikit-Learn
- Easy to implement and use: Naive Bayes classifier in scikit-learn is easy to implement and use, making it suitable for beginners and experts alike.
- Fast training and prediction: Naive Bayes classifier is known for its fast training and prediction times, making it efficient for large datasets.
- Handles high-dimensional data well: Naive Bayes classifier performs well on high-dimensional data, making it suitable for text classification and other applications with a large number of features.
- Handles missing values: Naive Bayes classifier in scikit-learn can handle missing values in the dataset, making it robust to incomplete data.
- Good performance with small datasets: Naive Bayes classifier performs well with small datasets, making it suitable for applications where data is limited.
- Interpretable results: Naive Bayes classifier provides interpretable results, making it easy to understand and explain the model's predictions.
- Can handle both binary and multiclass classification: Naive Bayes classifier in scikit-learn can handle both binary and multiclass classification problems, making it versatile for different types of tasks.
How-To Guide
- To create a Naive Bayes classifier using Scikit-learn, follow these steps:
- Install Scikit-learn: If you haven't already installed Scikit-learn, you can do so by running the following command:
- ```bash
- pip install scikit-learn
- ```
- Import the necessary libraries: In your Python script, import the required libraries for creating a Naive Bayes classifier:
- ```python
- from sklearn.naive_bayes import GaussianNB
- from sklearn.model_selection import train_test_split
- from sklearn.metrics import accuracy_score
- ```
- Load your dataset: Load your dataset into a pandas DataFrame or numpy array. Make sure to separate your features (X) and target variable (y).
- Split the dataset: Split your dataset into training and testing sets using the train_test_split function:
- ```python
- X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
- ```
- Create and train the Naive Bayes model: Create an instance of the GaussianNB class and fit the model to your training data:
- ```python
- nb = GaussianNB()
- nb.fit(X_train, y_train)
- ```
- Make predictions: Use the trained model to make predictions on the test data:
- ```python
- y_pred = nb.predict(X_test)
- ```
- Evaluate the model: Calculate the accuracy of the model by comparing the predicted values
Related Topics
Related Topics
- Loading related topics...
Conclusion
In conclusion, Naive Bayes classifier is a simple yet powerful algorithm for classification tasks, especially in text and sentiment analysis. The implementation of Naive Bayes in scikit-learn library makes it easy to use and apply in various machine learning projects. With its efficiency and effectiveness in handling large datasets, Naive Bayes in scikit-learn is a valuable tool for data scientists and machine learning practitioners. Its ability to quickly train and classify data makes it a popular choice for many real-world applications.
Similar Terms
- Naive Bayes algorithm
- Scikit-learn Naive Bayes
- Machine learning classification
- Python Naive Bayes
- Gaussian Naive Bayes
- Multinomial Naive Bayes
- Text classification
- Data science Naive Bayes
- Naive Bayes tutorial
- Naive Bayes implementation
More Articles
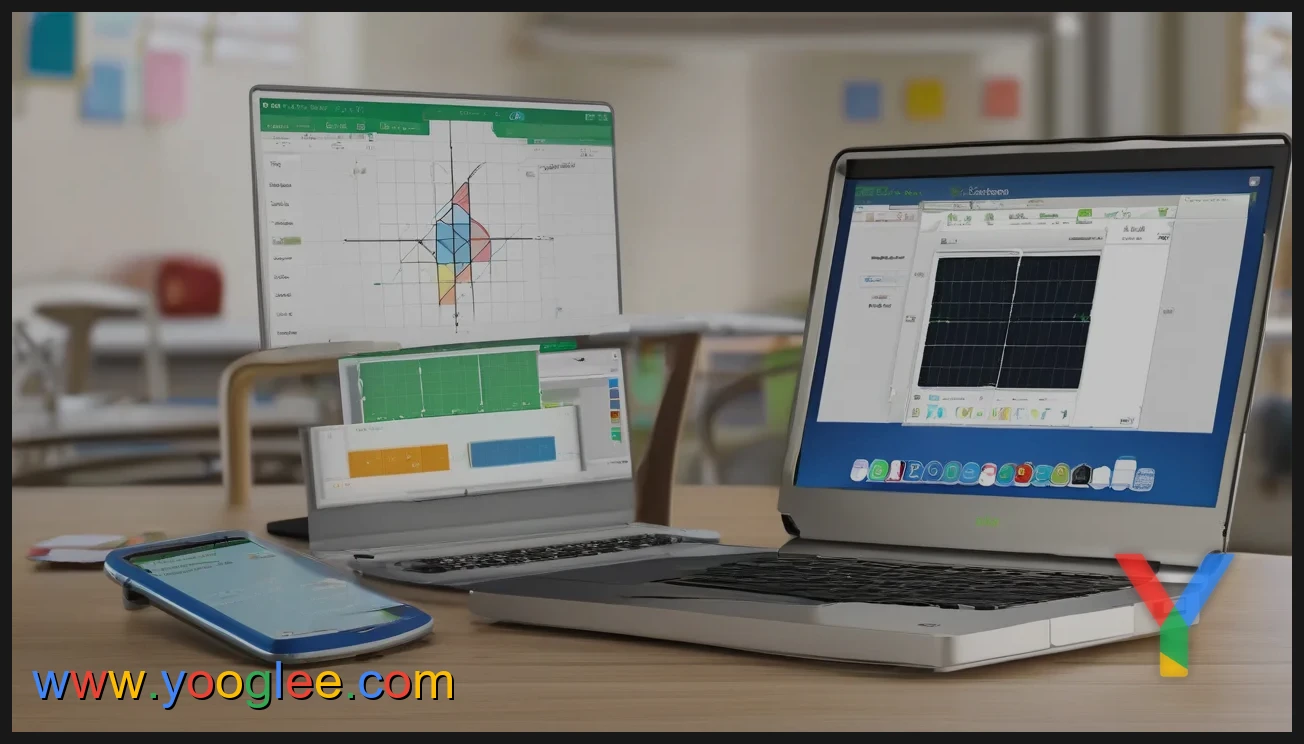
Exploring Desmos: A Collaborative Learning Journey
Join us on Desmos as we learn together and explore the world of math in a fun and interactive way. Get ready to collaborate, problem solve, and discover new concepts with our community of learners.
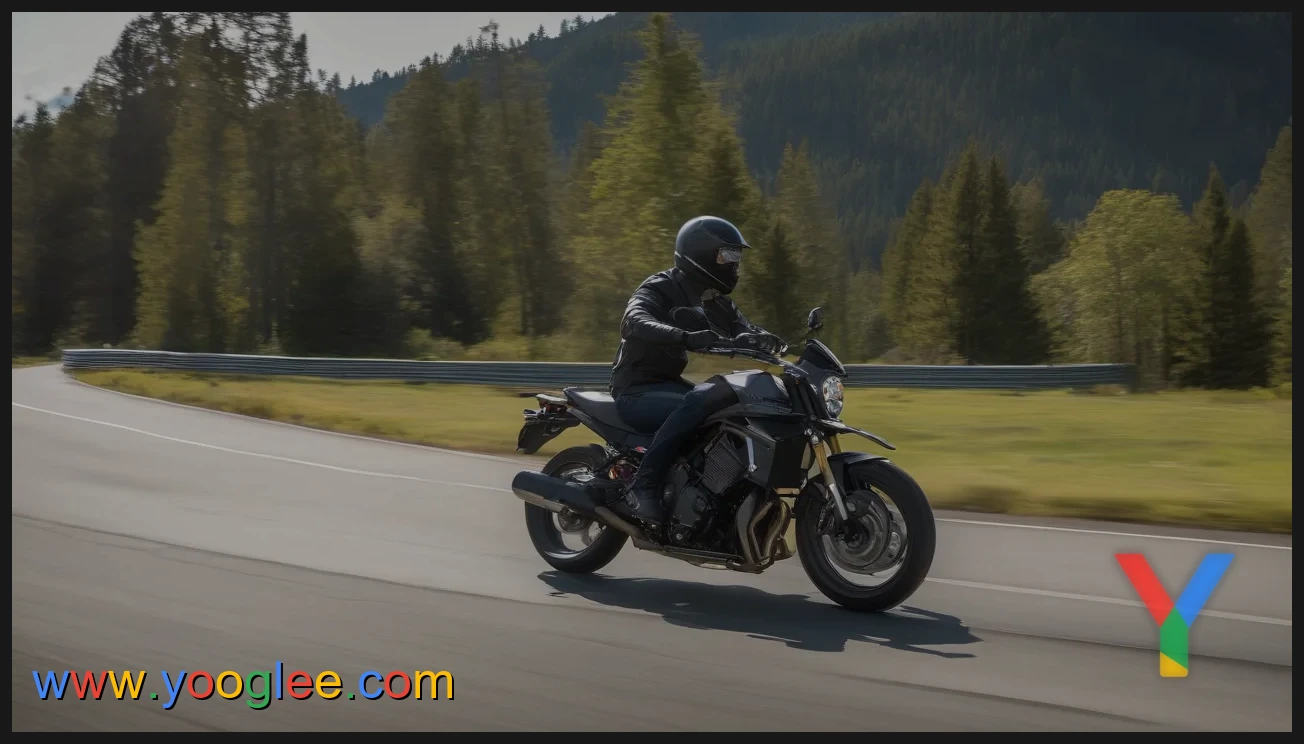
Mastering the Art of Motorcycle Riding: How Long Does it Take to Learn to Drive a Motorcycle?
Learn how long it typically takes to master the skills needed to drive a motorcycle, from basic controls to road safety, and become a confident rider.
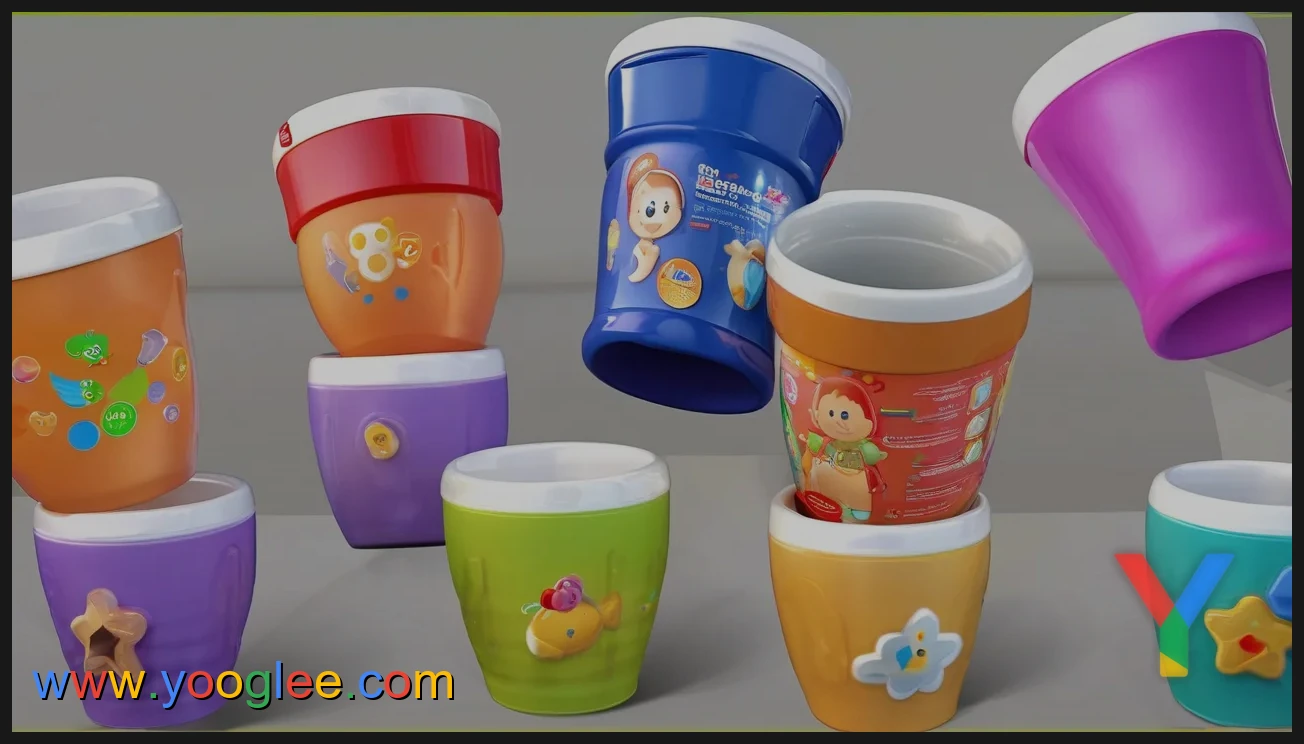
Fisher Price Laugh and Learn Cup: Interactive Toy for Baby\'s Development
Discover the Fisher Price Laugh and Learn Cup, a fun and interactive toy that helps babies learn while they play. With music, lights, and activities, this cup is sure to keep little ones entertained for hours.
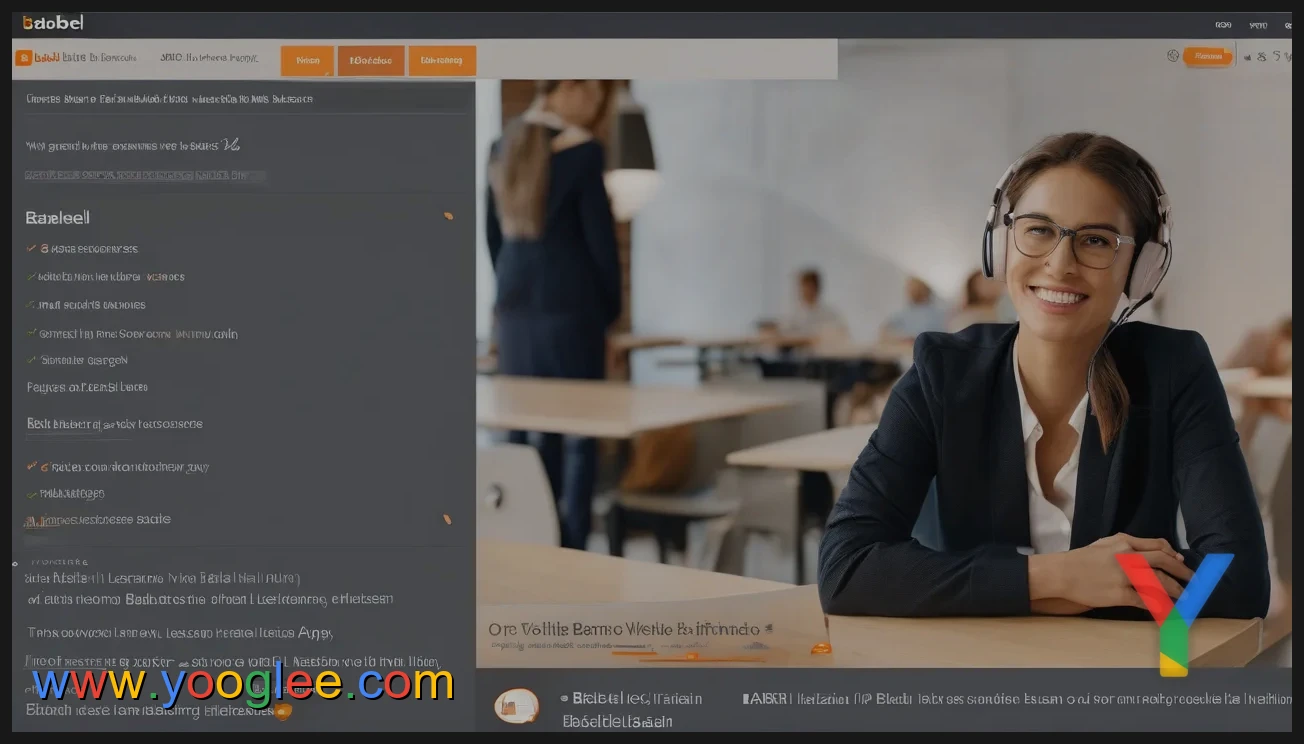
Babbel: Your Ultimate Guide to Learning Italian Quickly and Easily
Learn Italian with Babbel's interactive and engaging language learning platform. Start speaking Italian confidently with Babbel's proven methods and personalized lessons. Join millions of users worldwide and unlock your potential with Babbel.
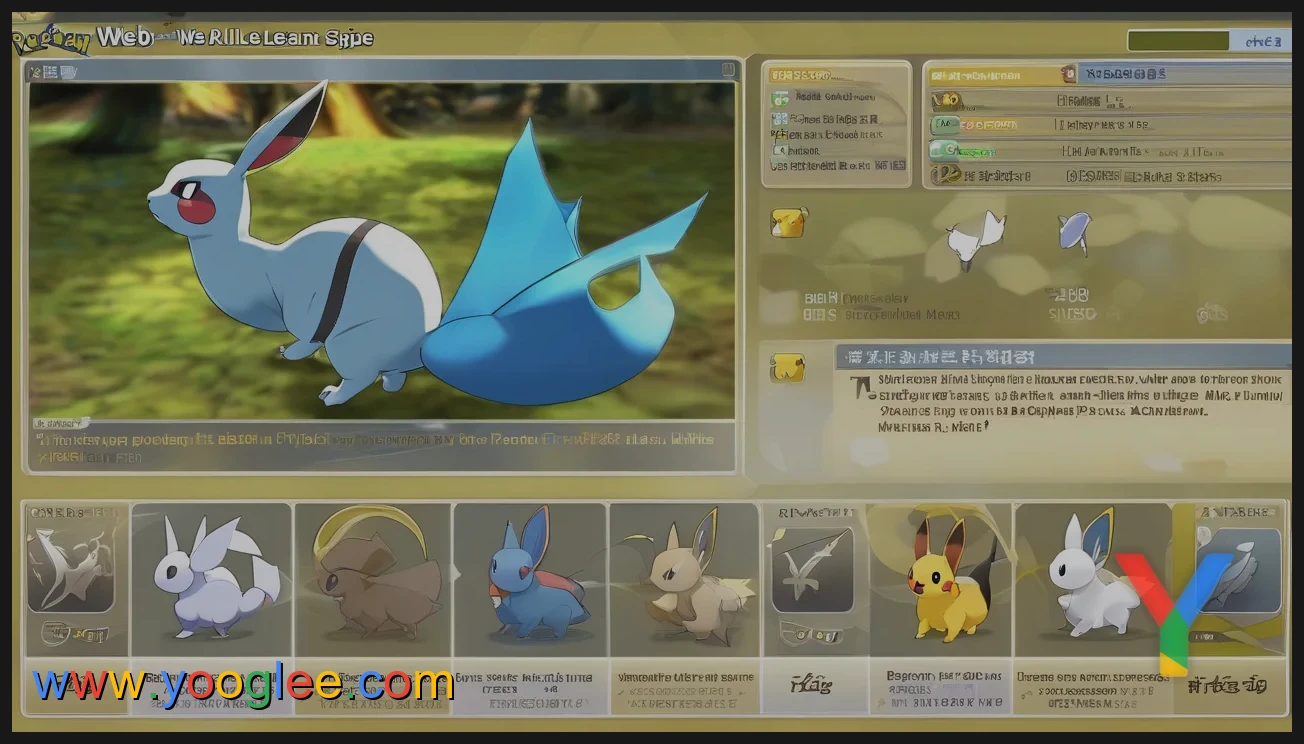
Complete List of Pokemon Capable of Learning False Swipe in Pokemon Games
Looking for a complete list of all Pokemon that can learn False Swipe? Look no further! Discover which Pokemon have the ability to use this essential move for catching and battling in the world of Pokemon.
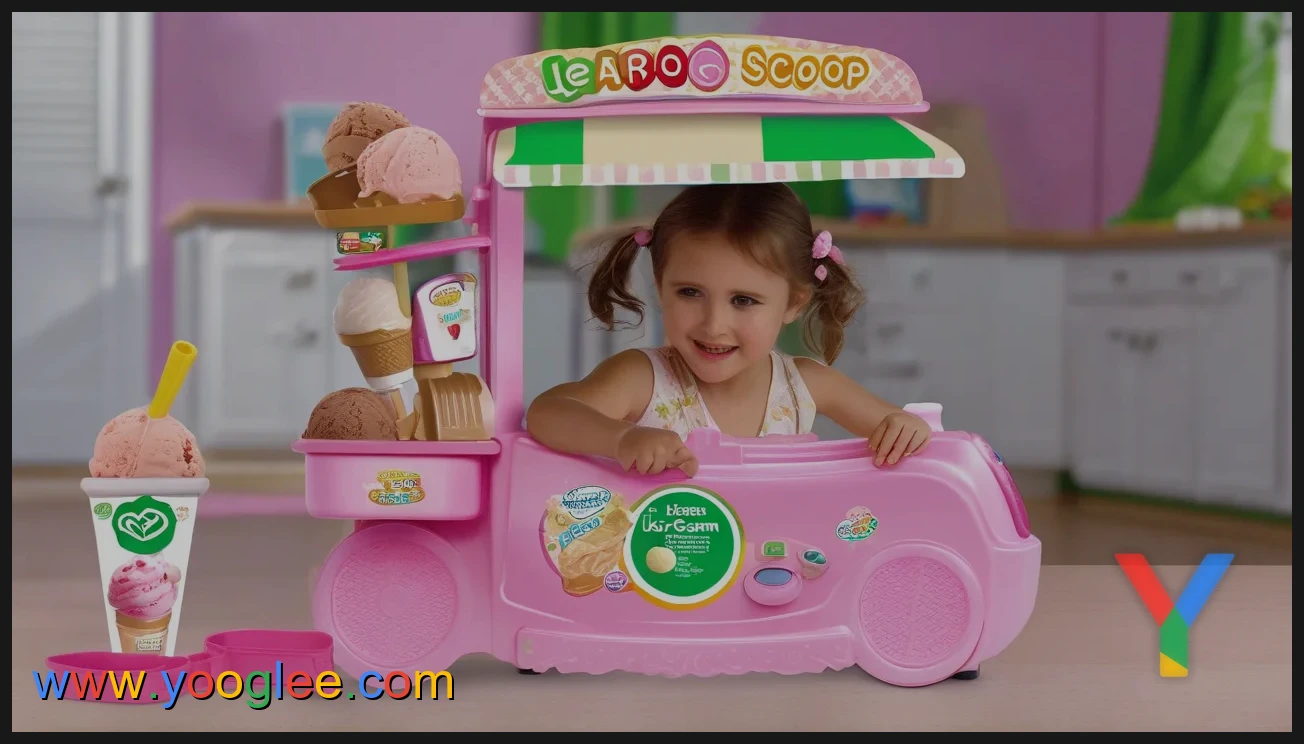
LeapFrog Scoop and Learn Ice Cream Cart Deluxe (Frustration-Free Packaging) - Pink: The Perfect Toy for Fun and Learning
Discover the ultimate playtime experience with the LeapFrog Scoop and Learn Ice Cream Cart Deluxe in pink, featuring frustration-free packaging. Your child will have endless fun learning and playing with this interactive toy!