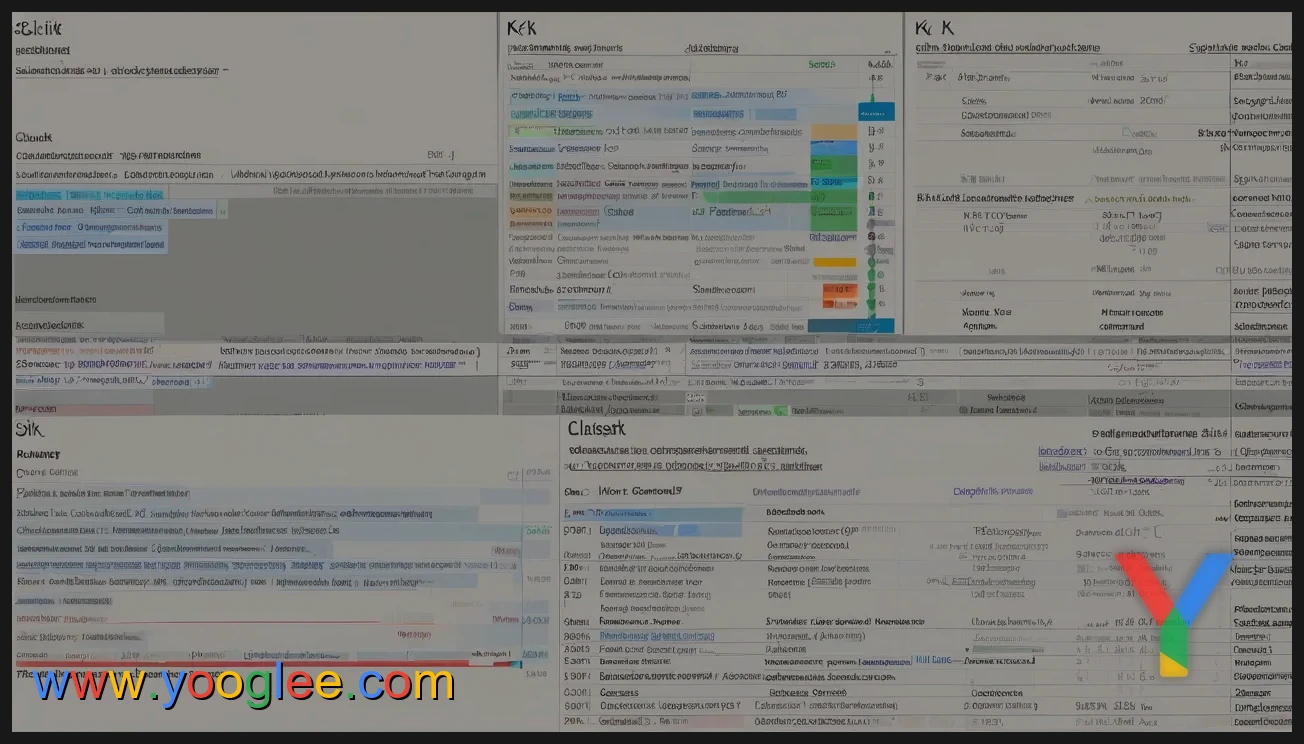
K Means Clustering with Scikit Learn
Published on 4/19/2025 • 5 min read
Implementation of k-means clustering using scikit-learn
K-means clustering is a popular unsupervised machine learning algorithm used for grouping data points into clusters based on their similarity. In this article, we will explore how to implement K-means clustering using the Scikit-learn library in Python. This powerful library provides a simple and efficient way to perform clustering tasks, making it a valuable tool for data analysis and pattern recognition. We will discuss the basic concepts of K-means clustering, how it works, and demonstrate how to use it to analyze and visualize data in real-world applications.
K-means clustering is a popular unsupervised machine learning algorithm used for clustering data points into groups based on similarity. The goal of K-means clustering is to partition data points into K clusters in such a way that each data point belongs to the cluster with the nearest mean. Scikit-learn is a powerful machine learning library in Python that provides a simple and efficient implementation of the K-means clustering algorithm. Using scikit-learn, you can easily apply K-means clustering to your dataset and analyze the resulting clusters. To perform K-means clustering using scikit-learn, you first need to import the KMeans class from the sklearn.cluster module. Next, you need to specify the number of clusters (K) that you want to create and fit the KMeans model to your data. Finally, you can use the predict method to assign each data point to a specific cluster and analyze the results. It is important to note that the performance of K-means clustering can be influenced by the initial placement of the cluster centroids. To address this issue, scikit-learn provides the k-means++ initialization method, which intelligently selects the initial centroids to improve the convergence of the algorithm. In conclusion, K-means clustering is a powerful technique for grouping data points into clusters based on similarity, and scikit-learn provides a user-friendly implementation of this algorithm. By leveraging the capabilities of scikit-learn, you can easily apply K-means clustering to your dataset and gain valuable
Benefits of K Means Clustering with Scikit Learn
- Easy to implement: K Means clustering algorithm is easy to implement using scikit learn library in Python.
- Scalability: K Means clustering is scalable to large datasets which makes it suitable for real-world applications.
- Fast and efficient: K Means clustering algorithm is computationally efficient and can handle large datasets with ease.
- Flexibility: K Means clustering allows you to specify the number of clusters you want to create, making it a flexible algorithm for different use cases.
- Interpretability: K Means clustering produces clusters that are easy to interpret and understand, making it useful for data analysis and visualization.
- Robustness: K Means clustering is robust to noise and outliers in the data, making it a reliable algorithm for clustering tasks.
- Versatility: K Means clustering can be applied to a wide range of data types and is suitable for various industries and domains.
How-To Guide
- K-means clustering is a popular unsupervised machine learning algorithm that groups data points into K clusters based on their similarities. In this guide, we will walk you through how to implement K-means clustering using the scikit-learn library in Python.
- Step 1: Install scikit-learn
- If you haven't already installed scikit-learn, you can do so using pip:
- ```bash
- pip install scikit-learn
- ```
- Step 2: Import the necessary libraries
- First, you need to import the required libraries:
- ```python
- import numpy as np
- from sklearn.cluster import KMeans
- ```
- Step 3: Prepare your data
- Next, you need to prepare your data for clustering. Make sure your data is in the form of a numpy array or pandas DataFrame.
- ```python
- data = np.array([[1, 2], [5, 8], [5, 8], [8, 8], [1, 0.6], [9, 11]])
- ```
- Step 4: Instantiate the KMeans class
- Now, you can instantiate the KMeans class with the desired number of clusters (K) and fit the model to your data.
- ```python
- kmeans = KMeans(n_clusters=2)
- kmeans.fit(data)
- ```
- Step 5: Get the cluster labels
- You can now get the cluster labels for each data point in your dataset.
- ```python
- labels = kmeans
Related Topics
Related Topics
- Loading related topics...
Conclusion
In conclusion, k-means clustering is a powerful unsupervised machine learning algorithm that can be easily implemented using the Scikit-learn library in Python. By grouping data points into clusters based on their similarities, k-means clustering can help identify patterns and relationships within datasets. With its flexibility and efficiency, k-means clustering is a valuable tool for various applications in data analysis, pattern recognition, and anomaly detection. By understanding the principles behind k-means clustering and utilizing the resources provided by Scikit-learn, researchers and practitioners can leverage this algorithm to gain valuable insights from their data.
Similar Terms
- K means clustering
- Scikit learn
- Clustering algorithms
- Unsupervised learning
- Python clustering
- Machine learning clustering
- Data clustering techniques
- K means clustering tutorial
- Scikit learn clustering example
- Clustering with scikit learn
More Articles
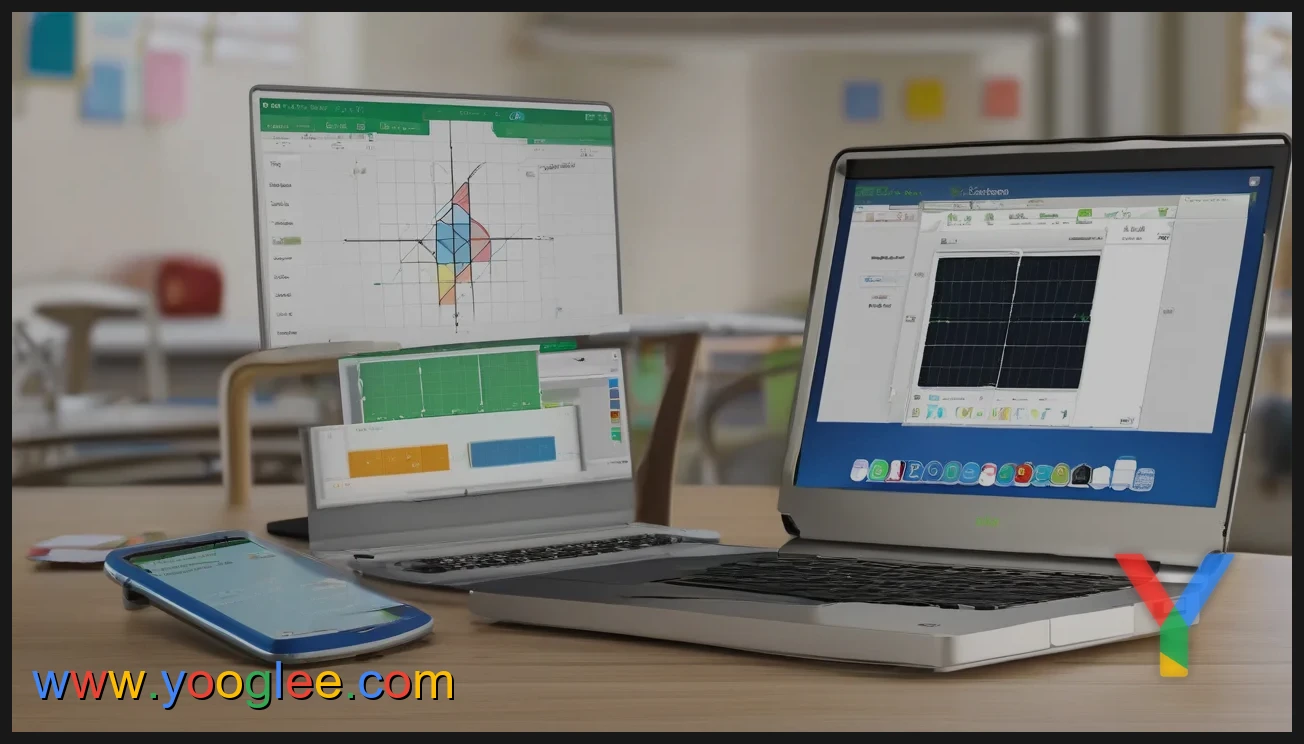
Exploring Desmos: A Collaborative Learning Journey
Join us on Desmos as we learn together and explore the world of math in a fun and interactive way. Get ready to collaborate, problem solve, and discover new concepts with our community of learners.
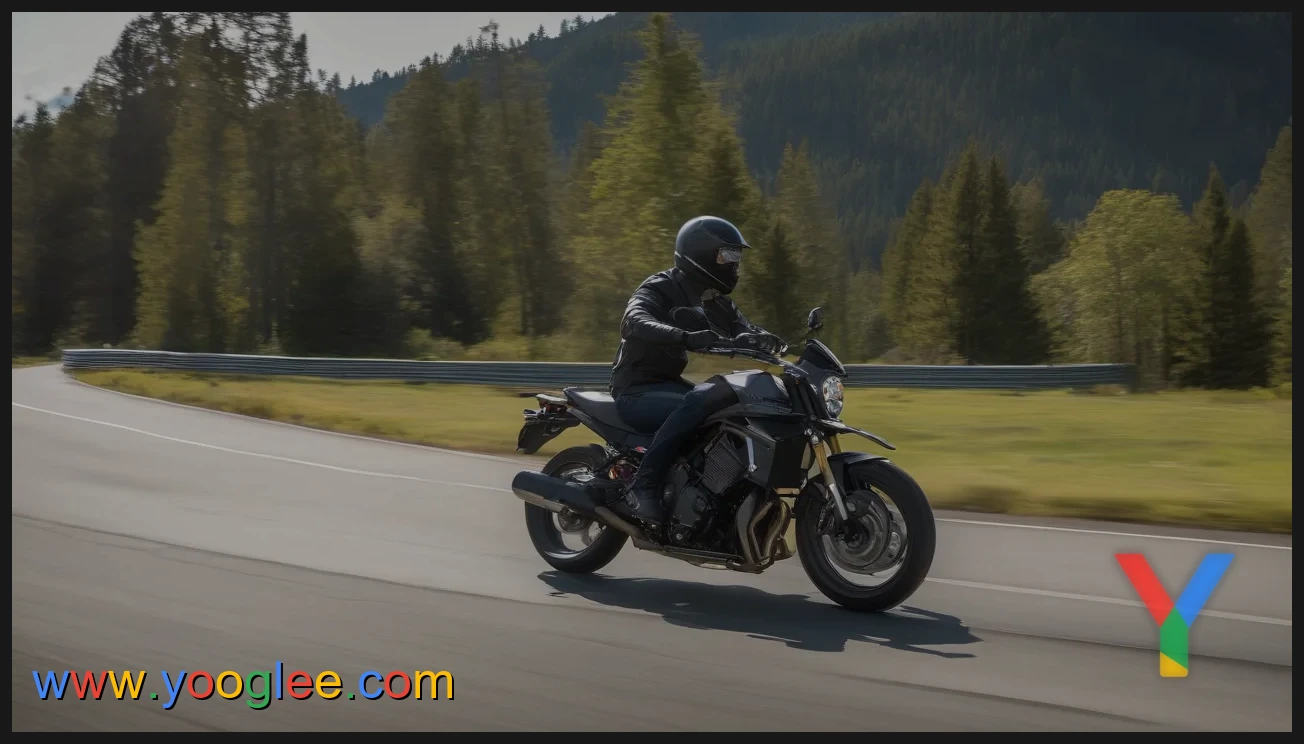
Mastering the Art of Motorcycle Riding: How Long Does it Take to Learn to Drive a Motorcycle?
Learn how long it typically takes to master the skills needed to drive a motorcycle, from basic controls to road safety, and become a confident rider.
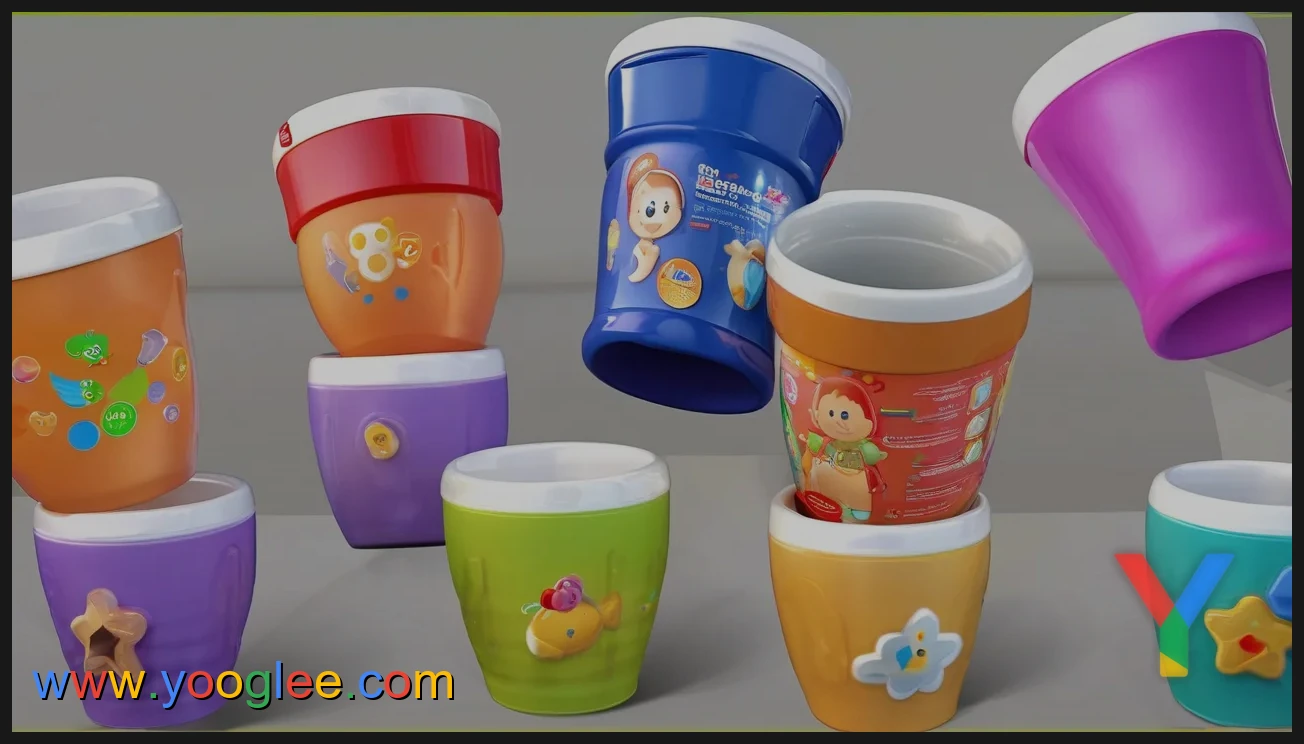
Fisher Price Laugh and Learn Cup: Interactive Toy for Baby\'s Development
Discover the Fisher Price Laugh and Learn Cup, a fun and interactive toy that helps babies learn while they play. With music, lights, and activities, this cup is sure to keep little ones entertained for hours.
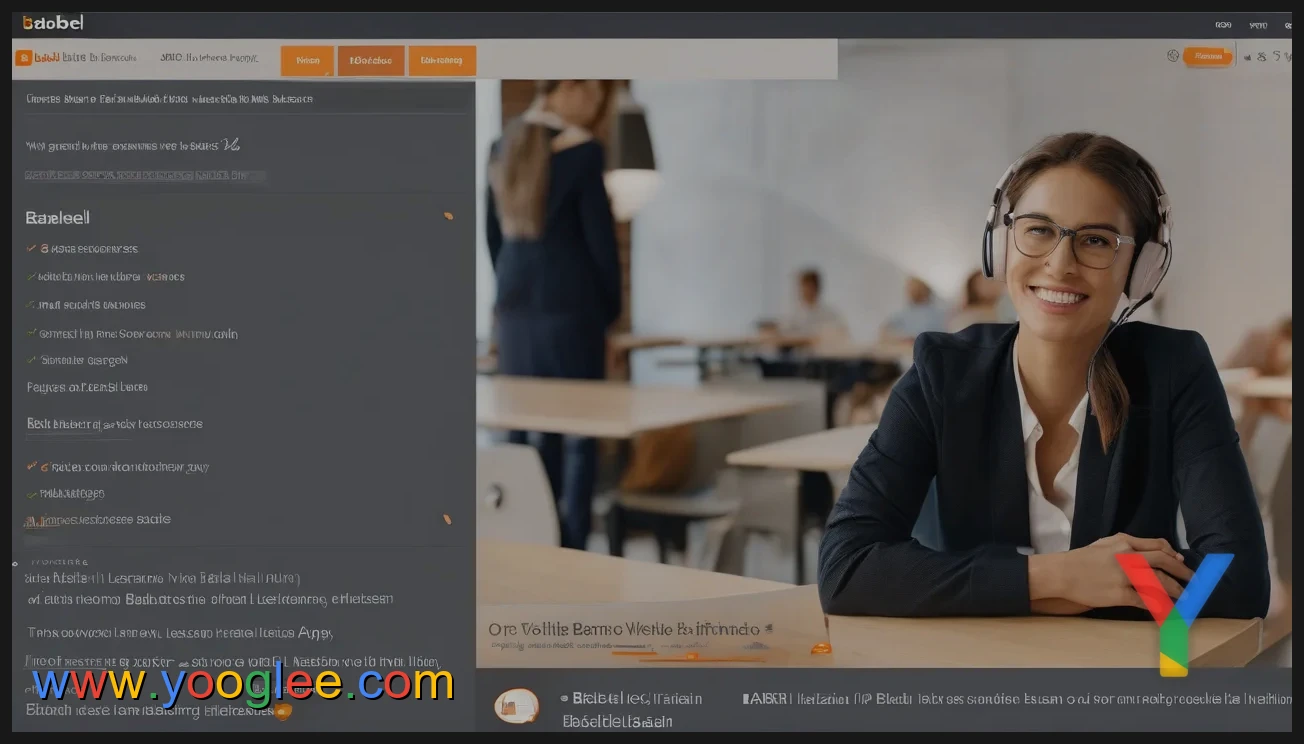
Babbel: Your Ultimate Guide to Learning Italian Quickly and Easily
Learn Italian with Babbel's interactive and engaging language learning platform. Start speaking Italian confidently with Babbel's proven methods and personalized lessons. Join millions of users worldwide and unlock your potential with Babbel.
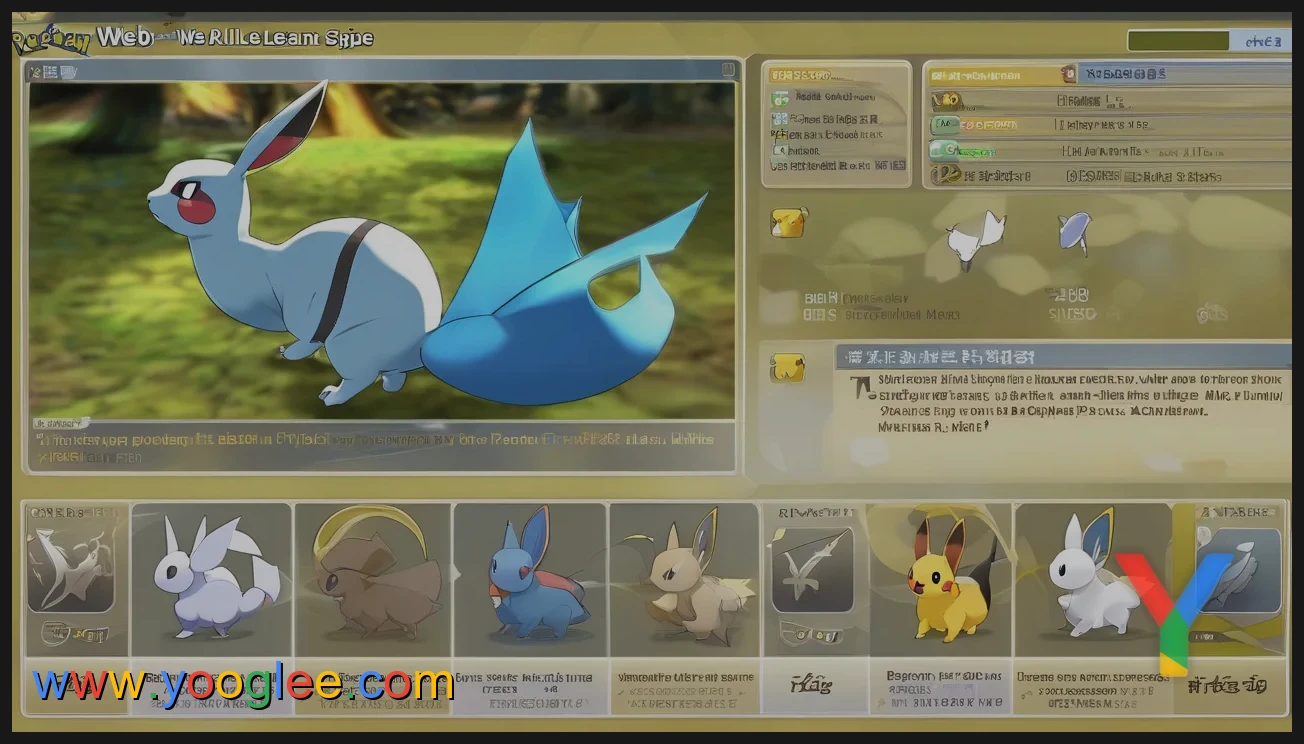
Complete List of Pokemon Capable of Learning False Swipe in Pokemon Games
Looking for a complete list of all Pokemon that can learn False Swipe? Look no further! Discover which Pokemon have the ability to use this essential move for catching and battling in the world of Pokemon.
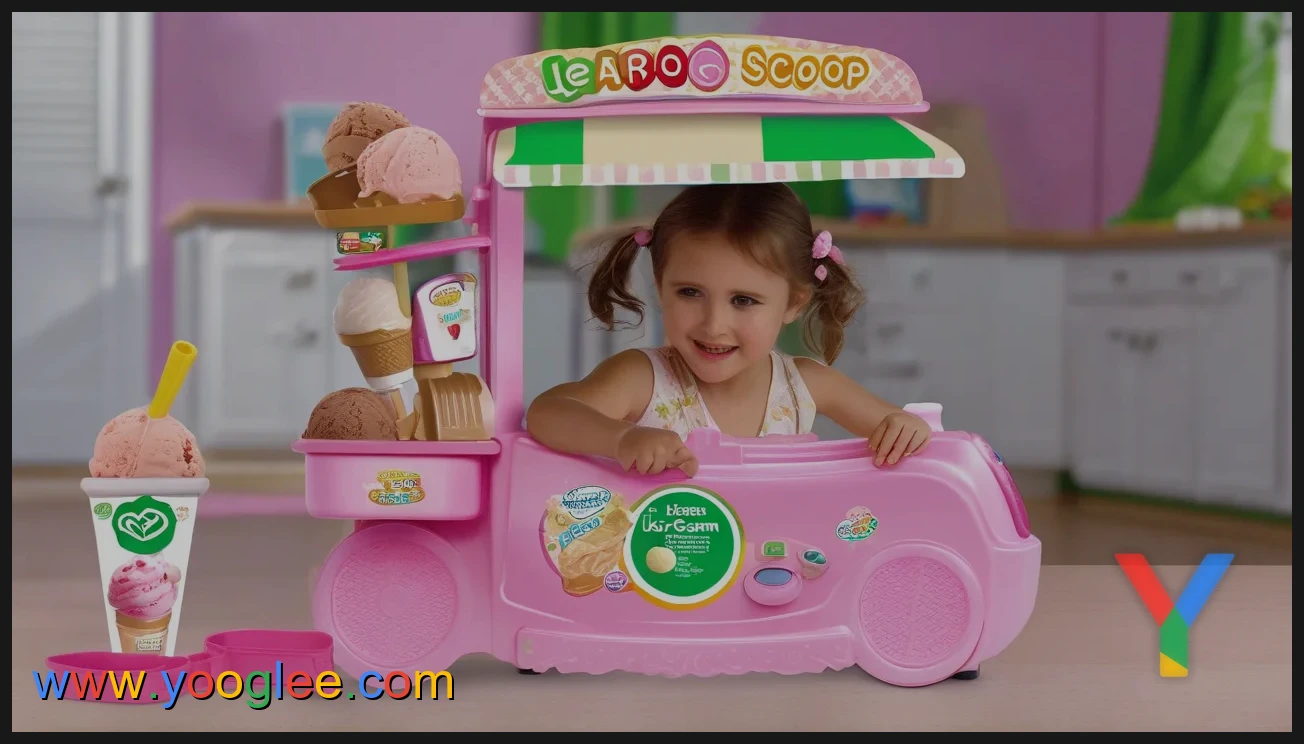
LeapFrog Scoop and Learn Ice Cream Cart Deluxe (Frustration-Free Packaging) - Pink: The Perfect Toy for Fun and Learning
Discover the ultimate playtime experience with the LeapFrog Scoop and Learn Ice Cream Cart Deluxe in pink, featuring frustration-free packaging. Your child will have endless fun learning and playing with this interactive toy!